Javascript is a web programming language that can be used to dynamically update the content of a web page. One of the many, many, dynamic tasks that can be performed with javascript is adding class names to HTML element. Dynamic manipulation of element classes provide a more dynamic web content views.
In this post, we will learn different approaches through which we can dynamically add class names to HTML element using javascript. The approach that you choose to use is matter of taste and convenience. We will take a step by step approach to discuss each method for a better understanding.
Table of Contents
- Add Class with classList Object
- Add Class with className Property
- Add Class with setAttribute() Method
- Conclusion
In the discussions that follow, we will assume the following HTML document in which we have defined three CSS styles.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style type="text/css">
.fs-40 {
font-size: 40px;
}
.italic {
font-style: italic;
}
.fw-700 {
font-weight: 700;
}
</style>
</head>
<body>
<div id="test">Apply CSS styles dynamically with javascript</div>
<script src="index.js"></script>
</body>
</html>
We will apply the CSS styles with classes .fs-40
, .fw-700
, and .italic
to the div
element dynamically using javascript. As indicated earlier, we will consider three different ways through which we can style the div
element using javascript. I will assume you are loading a script file with name index.js, as shown in line 25
of the HTML document. You can however choose to write your javascript code inline in the same HTML document file.
Add Class with classList
Object
The first method that we will use to add a class name to HTML element dynamically is the add()
method of classList
object. The classList
object maintains a list of class names for an HTML element. Having the class names of an element as a list provides an easy interface to remove or add additional class names to the element. We can add new class names to an element by calling the add()
method of the classList
object on the HTML element.
Using the HTML document shown earlier, let’s see how we can add class names to the div
element using javascript.
// get reference to the div element
const div = document.getElementById("test");
// add new class to the element
div.classList.add("fs-40");
In the above code, we obtain a reference to the div
element on line 2
. On line 5
, we use the classList
object of the div
element to call add()
by specifying the class name fs-40
. This applys a font-size: 40px
to the div
content. The result is shown in the following image:
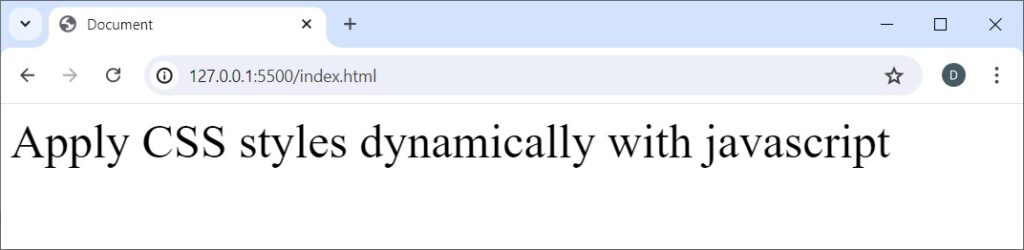
To add multiple class names in a single call, we will need to pass a comma-separated class names to the add()
method:
// get reference to the div element
const div = document.getElementById("info");
// add new class to the element
div.classList.add("fs-40", "italic");
The above code applies a font-size: 40px
and font-style: italic
to the div
element text with class names .fs-40
and .italic
respectively. The result is shown below:
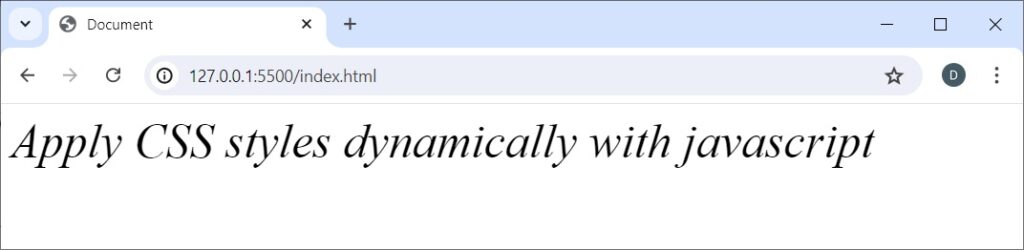
As can be seen, the div
element text has italic
font style applied with a font size of 40px
as defined in the document style specification.
Thus, we can use javascript to add class names to HTML elements by calling add()
method of the classList
object. We invoke classList
object with the HTML element that we want to apply the styles.
Add Class with className
Property
The className
property of an element is a getter and a setter property that is used to get and set the class attribute of an element respectively. This implies that we can use className
property to set a class name and also use it to get the class names that have been specified on an element. As className
is of type string
, we can add additional class names by concatenating the className
property with new class names. Let’s look at how we set and add class names to an element using the className
property.
We will begin by resetting our javascript code so that no styles are applied to the div
element text. A preview of the document as viewed in the browser without any styles applied is shown as follows:
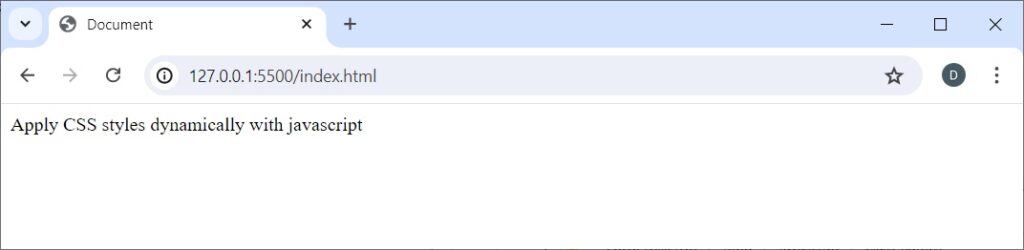
To set a class name on an element, we only need to set the name of the class that we want to apply by setting it to the className
property. An example is shown in the following javascript code:
// get the div element
const div = document.getElementById("test");
// set the size of the text to 40px
div.className = 'fs-40';
A view of document after adding the class name fs-40
is shown in the following image:

As indicated earlier, the className
property is of type string
. We can therefore add additional class names by concatenating the new class names to the className
property.
// get the div element
const div = document.getElementById("test");
// set the size of the text to 40px
div.className = 'fs-40';
// add the italic style to the existing style
div.className += ' italic';
When adding additional class names with string concatenation, it is helpful to include a space before the new class names being appended. Observe from line 8
that there is a space before the class name that is being append. That is: " italic"
. With className already containing fs-40
, the preceding space character results in the className
property to contain the value "fs-40 italic"
. If we omit the initial space before the new class name we want to add, then className
will contain the value "fs-40italic"
which isn’t what we want. In this case, we will even lose the style for the last added class name.
A view of the document with class names fs-40
and italic
added is shown as follows:
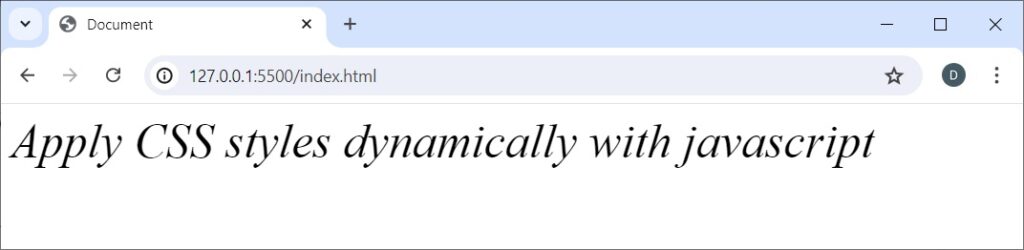
It is not necessary to first set an initial class name and later append remaining class names with className
property. In fact, we can apply string concatenation in our first step when no class names have initially been set. The result will be the same:
// get the div element
const div = document.getElementById("test");
// set the size of the text to 40px and apply italic font style
div.className += ' fs-40 italic';
Add Class with setAttribute Method
Another approach to set or update the class attribute of an element is to call setAttribute()
method of the target element. This is done by specifying class
as first argument, and the class names as the second argument.
// get the div element
const div = document.getElementById('test');
// set the class name with setAttribute
div.setAttribute('class', 'fs-10');
We are not restricted to setting single class names at a time with setAttribute()
. We can set multiple class names in a single call to setAttribute()
method:
// get the div element
const div = document.getElementById('test');
// set the class name with setAttribute
div.setAttribute('class', 'fs-10 italic fw-700');
Setting class names with setAttribute()
method will override any class names that were initially set. In order to append to existing class names that were previously set, we will need to get the existing class names by calling getAttribute()
method, and then concatenate the return value with the additional class names we want to append.
// get the div element
const div = document.getElementById("test");
// set the size of the text to 40px
div.setAttribute('class', 'fs-40');
// add the italic and font weight styles to the existing style
div.setAttribute('class', div.getAttribute('class') + ' italic fw-700');
If you are a lover of javascript template strings, that will also do fine, and the result will be the same:
// get the div element
const div = document.getElementById("test");
// set the size of the text to 40px
div.setAttribute('class', 'fs-40');
// add the italic and font weight styles to the existing style
div.setAttribute('class', `${div.getAttribute('class')} italic fw-700`);
Conclusion
Using the javascript programming language, we can dynamically apply CSS styles to HTML elements for dynamic web views. There are different ways through which this can be accomplished.
HTML elements contain the classList
object which provides methods for adding and removing CSS classes. The classList
object contains other methods for manipulating element styles. To add a class name to an element, we call the add()
method of the classList
object. We can add multiple class names in a single call by passing comma-separated class names to the add()
method:
// get reference to the div element
const div = document.getElementById("info");
// add new class to the element
div.classList.add("fs-40");
// add multiple classes in a single call
div.classList.add("fs-40", "italic");
Another approach that can be used to dynamically add class names with javascript is to set or append to the className property of the HTML element:
// get the div element
const div = document.getElementById("test");
// set the size of the text to 40px
div.className = 'fs-40';
When appending to existing class names with className
property, it is important to precede the new class names with a space. This is to avoid appending the new class name to the last added class name with no space between them:
// get the div element
const div = document.getElementById("test");
// set the size of the text to 40px
div.className = 'fs-40';
// add the italic style to the existing style
div.className += ' fw-700 italic';
Moreover, we can use javascript to dynamically add class names to an element by calling the setAttribute()
method of the HTML element. With this method, we specify the attribute name as class
by passing it as the irst argument, and then pass the class names that we want to set as the second argument:
// get the div element
const div = document.getElementById('test');
// set the class name with setAttribute
div.setAttribute('class', 'fs-10 fw-700');
If we need to add to existing class names, we can get the existing class names by calling getAttribute()
, and then append the new class names that we want to add:
// get the div element
const div = document.getElementById("test");
// set the size of the text to 40px
div.setAttribute('class', 'fs-40');
// add the italic and font weight styles to the existing style
div.setAttribute('class', div.getAttribute('class') + ' italic');